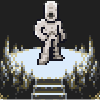
I need some help with an array
#1
Posted 15 December 2008 - 07:50 PM
and also, enemies Vx and Vy can be manipulated, correct?
#2
Posted 15 December 2008 - 08:14 PM
import "std.zh"
const int MAXENEMIES=100;
ffc script enemycontrol
{
void run()
{
npc e[MAXENEMIES];
Waitframes(4);
for(int i=0;i<Screen->NumNPCs() && i<MAXENEMIES;i++)
{
e[i]=Screen->LoadNPC(i);
}
//add rest of the script here
}
}
(Thanks goes to Gleeok for giving me the idea of actually doing this through a script posted at AGN.)
Edited by Elmensajero, 15 December 2008 - 08:19 PM.
#3
Posted 15 December 2008 - 08:32 PM
not as simple as i remember it being...
i just need it to find all the enemies and be able to edit their Vx and Vy...
and also, i need to put this into a global script.
I'm making a vaccuum-like (how the hell do you spell that word?) effect that pulls enemies into a black hole and kills the ones that actually get there.
#4
Posted 16 December 2008 - 04:35 AM
I'm also not sure whether you can pass even a constant as the size of an array, I'm pretty sure it has to be a number.
If you're doing it in the global script, you needn't really load them as an array, you only need one enemy pointer which you check all enemies with.
npc e = Screen->LoadNPC(i);
if(npc is close enough){//do some collision checking and movement routines here...
move npc over;
}
}
I've got a code that sucks things in at home aswell if you want (wrote it for Kirby), but I can't get hold of it at the moment cause I'm at school.
Enemies don't have Vx and Vy values that you can manipulate easily like ffcs I'm afraid.
#5
Posted 16 December 2008 - 10:47 AM
i do need a vaccuum, but i need it to act a certain way.
when the enemies are farther away from it, it pulls them quickly, and the closer they get, the slower they pull.
and i thought i could manage this with the s=d/t formula... i noticed that if you put an ffc's coordinates in, it would achieve that effect because it checks every frame for the ffc's coordinates and therefore changes the speed of its movement.
but like you just said, that cant be done with enemies... so could i have a look at your vaccuum? please? lol
#6
Posted 16 December 2008 - 11:25 AM
int SuctionRange = 48;
for(i=1;i<=Screen->NumNPCs();i++){
npc e = Screen->LoadNPC(i);
if((Distance(Link->X,Link->Y,e->X,e->Y) <= SuctionRange){
int m;
if(Link->X-e->X != 0) m = (Link->Y-e->Y)/(Link->X-e->X);
if(Link->X-e->X > 0) e->X += SuctionSpeed;
else e->X = e->X -= SuctionSpeed;
int c = e->Y-m*e->X;
e->Y = m*e->X+c;
}
}
That code goes through all enemies on the screen, checks whether they are within 'SuctionRange' pixels of Link, and then moves them over to him at a rate of 'SuctionSpeed' pixels per frame.
If you want to alter the speed they're moved at dependant on the distance, all you'd have to do is have a bit of code to change SuctionSpeed depending on how far away the enemies are.
Obviously if you want to change where they're sucked to from Link to something else, just change the Link->X and Link->Y to the coordinates.
Good to see you back by the way.
Where did you go?
#7
Posted 16 December 2008 - 11:41 AM
so i had to get a new one, and here i am with vista. works better than i had expected...
but yeah, thanks for this piece of code =) ill be sure to post a demo quest of what im using this for, if it works. (knowing my scripting luck, it wont but still)
thanks. i really appreciate it. and its good to see you again.
EDIT: and suction speed is in pixels per frame, correct?
Edited by Master Maniac, 16 December 2008 - 11:43 AM.
#8
Posted 16 December 2008 - 12:15 PM
Also, I just realised it won't work if the enemy is directly to the above or below Link (at the same Y position), due to divide by zero.
I can fix that if you want.
Moved to Scripting Practise.
#9
Posted 16 December 2008 - 12:24 PM
anyway, yes that would be nice joe, adding the suction from directly above and below.
I'm almost done with the script. just have to add in the FFC setup for it and im done.
here is what i got so far:
//lets see if I can do this properly again... this shouldnt be too difficult now...
//also thanks to joe for the help with this script.
//VARIABLES!!
int blackholetile;
//thats all ill do for now... this is just for warmup so i dont want to do anything too complex.
bool blackhole=false;
item script blackholewand(){
blackhole=true;
}
//global part of the script below.
if(blackhole){
int seconds_exist= 5;
int SuctionSpeed = 2;
int SuctionRange = 48;
int frames_exist=seconds_exist*60;
int exist;
bool blackhole_existing;
blackhole_cast=Screen->LoadFFC(1);
if(!blackhole_existing){
exist=0;
}
if(Link->Dir=DIR_UP){
blackhole_cast->X=Link->X;
blackhole_cast->Y=Link->Y-3;
blackhole=false;
blackhole_existing=true;
}
if(Link->Dir=DIR_DOWN){
blackhole_cast->X=Link->X;
blackhole_cast->Y=Link->Y+3;
blackhole=false;
blackhole_existing=true;
}
if(Link->Dir=DIR_LEFT){
blackhole_cast->X=Link->X-3;
blackhole_cast->Y=Link->Y;
blackhole=false;
blackhole_existing=true;
}
if(Link->Dir=DIR_RIGHT){
blackhole_cast->X=Link->X+3;
blackhole_cast->Y=Link->Y;
blackhole=false;
blackhole_existing=true;
}
while(blackhole_existing){
exist++;
if(exist=frames_exist){
blackhole_exist=false;
}
for(i=1;i<=Screen->NumNPCs();i++){
npc e = Screen->LoadNPC(i);
if((Distance(blackhole_cast->X,blackhole_cast->Y,e->X,e->Y) <= SuctionRange){
int m;
if(blackhole_cast->X-e->X != 0) m = (blackhole_cast->Y-e->Y)/(blackhole_cast->X-e->X);
if(blackhole_cast->X-e->X > 0) e->X += SuctionSpeed;
else e->X = e->X -= SuctionSpeed;
int c = e->Y-m*e->X;
e->Y = m*e->X+c;
if(e->X>=blackhole_cast->X-8&&e->X<=blackhole_cast->X+8&&e->Y>=blackhole_cast->Y-8&&e->Y<=blackhole_cast->Y+8){
e->HP=0;
}
}
}
i know i know its not perfect, and its kinda buggy, but i wanted to do something small before I got into quest design again.
eventually it will have the screen quake and everything and it will have a better capatability with larger that 16x16 sprites for black holes lol
but this is the best i got so far. I'm pretty sure im missing some vital stuff... but im back to the nooblet level of scripting until i get back into the swing of things
#10
Posted 16 December 2008 - 12:35 PM

Anyways MM, I made a script that fires a 2x2 whirlwind that sucks in enemies as it moves across the screen a while ago. Take a look at it, or feel free to use it.
Actually, I like Elemensajo's method because the pointers stay valid. You just add Screen->LoadNPC(i+1); to it.
#11
Posted 16 December 2008 - 12:39 PM
And I've just realised how convoluted that sounded >_<
I'll go fix it myself if you don't get it.
You need 'void run' inside the item script, and obviously your global section isn't actually part of the global script yet.
Be careful with your '=' is set to and '==' is equal to.
I'm not sure if the link-up between the item script and global script is quite right, but I haven't looked too hard so it might be.
EDIT:
I know that's all you have to do to fix it.
It's not really all that applicable to the global script though, unless you set up something to check whether you've changed screens or not.
Then you need to re-load the array every time you change screens, and (if you want to do it properly) if the number of enemies on the screen increases.
Having an array is great for an ffc script, but it's less useful in the global script.
#12
Posted 16 December 2008 - 12:41 PM
where is that script, gleeok? my black hole is supposed to be stationary, but that would be nice to look at as a refrence
EDIT: ahh forgot the = and == difference. thanks for pointing that out lol
Edited by Master Maniac, 16 December 2008 - 12:43 PM.
#14
Posted 16 December 2008 - 12:57 PM
there are only 14 posts here...
(pardon me for my stupidity right now. its my medication.)
1 user(s) are reading this topic
0 members, 1 guests, 0 anonymous users