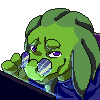
Energy Ball tennis
Started by
Smiles the Death Bringer
, Jul 25 2010 01:46 PM
7 replies to this topic
#1
Posted 25 July 2010 - 01:46 PM
Does anyone have a script for something like the final boss of OoT where you toss the energy ball thing back and forth
#2
Posted 25 July 2010 - 02:49 PM
I wrote one a while back, it worked pretty well last time I checked. What do you want the script to do specifically? Do you want sound effects when you hit it, or when the energy ball hits the boss? Warping after the boss is hit, choosing for the energy ball to travel horizontally or vertically?
Just trying to get an idea of what you want. My script works, but there could be some tweaks it doesn't have.
Just trying to get an idea of what you want. My script works, but there could be some tweaks it doesn't have.
#3
Posted 25 July 2010 - 04:38 PM
I want it to shoot out of an object you hit it with the sword then it goes back and hits the object and deactivates it
#4
Posted 26 July 2010 - 07:28 PM
CODE
import "std.zh"
//Energy ball ID
const int EW_ENERGYBALL = EW_SCRIPT1;
const int LW_ENERGYBALL = LW_SCRIPT1;
//Energy ball collision detection
const int ENERGY_BALL_SIZE = 16;
//Graphics data
const int ENERGYBALL_ORIGINALTILE = 0;
const int ENERYBALL_NUMFRAMES = 4;
const int ENERGYBALL_CSET = 6;
const int ENERGYBALL_ASPEED = 5;
float InvertAngle(float radians)
{
radians -= PI;
radians = WrapAngle(radians);
return radians;
}
eweapon Create_EnergyBall(int x1,int y1,int x2,int y2,float step)
{
eweapon ball = CreateEWeaponAt(EW_ENERGYBALL,x1,y1);
//setting its direction
ball->Angular = true;
ball->Angle = RadianAngle(x1,y1,x2,y2);
//setting its speed
ball->Step = step;
//setting its hitbox
ball->HitWidth = ENERGY_BALL_SIZE;
ball->HitHeight = ball->HitWidth;
//Setting the graphics
ball->TileWidth = 1;
ball->TileHeight = 1;
ball->ASpeed = ENERGYBALL_ASPEED;
ball->OriginalCSet = ENERGYBALL_CSET;
ball->NumFrames = ENERGYBALL_NUMFRAMES;
ball->OriginalTile = ENERGYBALL_ORIGINALTILE;
//return the ball
return ball;
}
//check if the ball touch a sword weapon, then invert the enemy weapon to a link weapon if it's the case
lweapon Invert_EnergyBall(eweapon ewep, int x2, int y2, float step)
{
//Checking if a sword collide with the ball
bool collide = false;
for(int i = 1; i <= Screen->GetNumLWeapons();i++)
{
lweapon sword = Screen->LoadLWeapon(i);
if(sword->ID == LW_SWORD && Collision(sword,ewep))
{
collide = true;
break;
}
}
//the ball variable
lweapon ball;
//if the sword collision was effective, it makes the eweapon not valid and creates a new lweapon
//its direction will be inverted from the eweapon's direction
if(collide)
{
ball = CreateLWeaponAt(LW_ENERGYBALL,ewep->X,ewep->Y);
//Setting the collision rectangle
ball->HitWidth = ewep->HitWidth;
ball->HitHeight = ewep->HitHeight;
//Setting the graphics
ball->TileWidth = 1;
ball->TileHeight = 1;
ball->OriginalTile = ewep->OriginalTile;
ball->OriginalCSet = ewep->OriginalCSet;
ball->NumFrames = ewep->NumFrames;
ball->ASpeed = ewep->ASpeed;
//Setting the movement
ball->Step = step;
ball->Angle = ewep->Angle;
//killing the eweapon
ewep->DeadState = WDS_DEAD;
//Inverting the angle
ball->Angle = InvertAngle(ball->Angle);
//returning a valid lweapon pointer
return ball;
}
//else it return a lweapon that is not valid. check for the value of lweapon->isValid() and do not delete the eweapon
else
{
//making sure that the lweapon is not valid
ball = CreateLWeaponAt(LW_ENERGYBALL,500,500);
ball->DeadState = WDS_DEAD;
//returns an invalid lweapon pointer
return ball;
}
}
//Simple example on how to use it
//
//The first enemy in the enemy list trow one ball and once it died it recreate an another one and so on
//
ffc script npc_trowBall
{
void run()
{
//The energy ball variable
eweapon energy_ball;
//The npc variable
npc enemy = ScreenLoadNPC(1);
while(true)
{
if( NumLWeaponOf(LW_ENERGYBALL)== 0 && NumEWeaponOf(EW_ENERGYBALL) == 0 )
energy_ball = Create_EnergyBall(enemy->X,enemy->Y,Link->X,Link->Y,300);
//Checking for energy balls
for(int i = 1; i <= Screen->NumEWeapons();i++)
{
eweapon ewep = Screen->LoadEWeapon(i);
if(ewep->ID == EW_ENERGYBALL)
{
lweapon new = Invert_EnergyBall(ewep,enemy->X,enemy->Y,300);
}
}
Waitframe();
}
}
}
//Energy ball ID
const int EW_ENERGYBALL = EW_SCRIPT1;
const int LW_ENERGYBALL = LW_SCRIPT1;
//Energy ball collision detection
const int ENERGY_BALL_SIZE = 16;
//Graphics data
const int ENERGYBALL_ORIGINALTILE = 0;
const int ENERYBALL_NUMFRAMES = 4;
const int ENERGYBALL_CSET = 6;
const int ENERGYBALL_ASPEED = 5;
float InvertAngle(float radians)
{
radians -= PI;
radians = WrapAngle(radians);
return radians;
}
eweapon Create_EnergyBall(int x1,int y1,int x2,int y2,float step)
{
eweapon ball = CreateEWeaponAt(EW_ENERGYBALL,x1,y1);
//setting its direction
ball->Angular = true;
ball->Angle = RadianAngle(x1,y1,x2,y2);
//setting its speed
ball->Step = step;
//setting its hitbox
ball->HitWidth = ENERGY_BALL_SIZE;
ball->HitHeight = ball->HitWidth;
//Setting the graphics
ball->TileWidth = 1;
ball->TileHeight = 1;
ball->ASpeed = ENERGYBALL_ASPEED;
ball->OriginalCSet = ENERGYBALL_CSET;
ball->NumFrames = ENERGYBALL_NUMFRAMES;
ball->OriginalTile = ENERGYBALL_ORIGINALTILE;
//return the ball
return ball;
}
//check if the ball touch a sword weapon, then invert the enemy weapon to a link weapon if it's the case
lweapon Invert_EnergyBall(eweapon ewep, int x2, int y2, float step)
{
//Checking if a sword collide with the ball
bool collide = false;
for(int i = 1; i <= Screen->GetNumLWeapons();i++)
{
lweapon sword = Screen->LoadLWeapon(i);
if(sword->ID == LW_SWORD && Collision(sword,ewep))
{
collide = true;
break;
}
}
//the ball variable
lweapon ball;
//if the sword collision was effective, it makes the eweapon not valid and creates a new lweapon
//its direction will be inverted from the eweapon's direction
if(collide)
{
ball = CreateLWeaponAt(LW_ENERGYBALL,ewep->X,ewep->Y);
//Setting the collision rectangle
ball->HitWidth = ewep->HitWidth;
ball->HitHeight = ewep->HitHeight;
//Setting the graphics
ball->TileWidth = 1;
ball->TileHeight = 1;
ball->OriginalTile = ewep->OriginalTile;
ball->OriginalCSet = ewep->OriginalCSet;
ball->NumFrames = ewep->NumFrames;
ball->ASpeed = ewep->ASpeed;
//Setting the movement
ball->Step = step;
ball->Angle = ewep->Angle;
//killing the eweapon
ewep->DeadState = WDS_DEAD;
//Inverting the angle
ball->Angle = InvertAngle(ball->Angle);
//returning a valid lweapon pointer
return ball;
}
//else it return a lweapon that is not valid. check for the value of lweapon->isValid() and do not delete the eweapon
else
{
//making sure that the lweapon is not valid
ball = CreateLWeaponAt(LW_ENERGYBALL,500,500);
ball->DeadState = WDS_DEAD;
//returns an invalid lweapon pointer
return ball;
}
}
//Simple example on how to use it
//
//The first enemy in the enemy list trow one ball and once it died it recreate an another one and so on
//
ffc script npc_trowBall
{
void run()
{
//The energy ball variable
eweapon energy_ball;
//The npc variable
npc enemy = ScreenLoadNPC(1);
while(true)
{
if( NumLWeaponOf(LW_ENERGYBALL)== 0 && NumEWeaponOf(EW_ENERGYBALL) == 0 )
energy_ball = Create_EnergyBall(enemy->X,enemy->Y,Link->X,Link->Y,300);
//Checking for energy balls
for(int i = 1; i <= Screen->NumEWeapons();i++)
{
eweapon ewep = Screen->LoadEWeapon(i);
if(ewep->ID == EW_ENERGYBALL)
{
lweapon new = Invert_EnergyBall(ewep,enemy->X,enemy->Y,300);
}
}
Waitframe();
}
}
}
The code include an exemple on how to use it. Didn't test it, I will do later.

If you want the enemy to knock back the energy ball, just ask.

Edited by lucas92, 29 July 2010 - 06:06 PM.
#5
Posted 29 July 2010 - 05:41 PM
umm what enemy and/or object throws this (I'm new to scripts) also thanks
#6
Posted 29 July 2010 - 06:03 PM
It can be any object or enemy. For example, a wall shooting the energy ball:
Make sure to include the previous code to make this work. btw, all the constants are meant to be changed, based on the tileset you're using and your preferences.
ps. This is some complex scripting I'm doing here so this might be normal to not understand the previous code and this one...
If you're new to scripting, you should go learn it here, otherwise you will get confused with my code:
http://www.purezc.co...showtopic=38176
CODE
//D0-D1 : The ball spawning location
ffc script wall_trowball
{
void run(int x,int y)
{
//the eweapon pointer
eweapon ewep;
//the lweapon pointer
lweapon lwep;
while(true)
{
//If there's no Energy ball
if(NumLWeaponsOf(LW_ENERGYBALL) == 0 && NumEWeaponsOf(EW_ENERGYBALL) == 0 )
{
//Then creates a energy ball that will track Link
ewep = Create_EnergyBall(x,y,Link->X,Link->Y,300);
}
//converting to lweapon, it will not return a valid one if it hasn't touch Link's sword
//if valid, it will track where it has been shot from
//This has to be called for every frame
lwep = Invert_EnergyBall(ewep,x,y,300);
// necessary or else it will cause the program to freeze
Waitframe();
}
}
}
ffc script wall_trowball
{
void run(int x,int y)
{
//the eweapon pointer
eweapon ewep;
//the lweapon pointer
lweapon lwep;
while(true)
{
//If there's no Energy ball
if(NumLWeaponsOf(LW_ENERGYBALL) == 0 && NumEWeaponsOf(EW_ENERGYBALL) == 0 )
{
//Then creates a energy ball that will track Link
ewep = Create_EnergyBall(x,y,Link->X,Link->Y,300);
}
//converting to lweapon, it will not return a valid one if it hasn't touch Link's sword
//if valid, it will track where it has been shot from
//This has to be called for every frame
lwep = Invert_EnergyBall(ewep,x,y,300);
// necessary or else it will cause the program to freeze
Waitframe();
}
}
}
Make sure to include the previous code to make this work. btw, all the constants are meant to be changed, based on the tileset you're using and your preferences.
ps. This is some complex scripting I'm doing here so this might be normal to not understand the previous code and this one...
If you're new to scripting, you should go learn it here, otherwise you will get confused with my code:
http://www.purezc.co...showtopic=38176
Edited by lucas92, 29 July 2010 - 06:05 PM.
#7
Posted 01 October 2010 - 09:28 AM
Well I'm a fast learner so I think I know what constants to change w/o the walkthrough thx
Ok do I use both the scripts separately or together
Well I kept testing it in different ways and none of them work
Ok do I use both the scripts separately or together
Well I kept testing it in different ways and none of them work
Edited by Ovasity, 01 October 2010 - 09:18 AM.
#8
Posted 01 October 2010 - 12:02 PM
The main part of the first script (up to the one labeled FFC script ___) is required for everything. The rest is just a customized FFC or two. Make your script with the functions of the first script and the FFC of whatever you choose.
1 user(s) are reading this topic
0 members, 1 guests, 0 anonymous users