Screens:
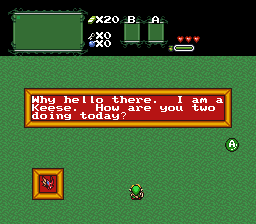
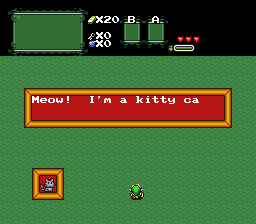
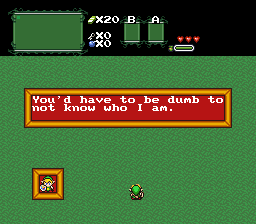
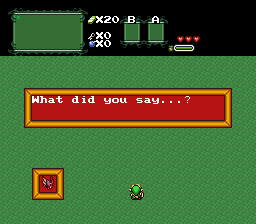
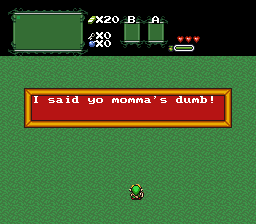
How do you work it? Well, it involves several things: A FFC, a list of combos for your characters, an array of integers for the sprite's cset, an item counter, a dummy item, and, of course, the script.
Don't worry, there's a step-by-step process to using it. Here's the code:
// Script to Manage Character Sprites During Cutscenes
// By LinktheMaster
import "std.zh"
// Int for the cset in which sprites use
int charCSet[50];
// Script to initialize the csets
global script init
{
void run()
{
// Sets the cset of the sprites
charCSet[0] = 7;
charCSet[1] = 8;
charCSet[2] = 7;
charCSet[3] = 2;
}
}
// Script for manages string char sprites
ffc script manageString
{
void run()
{
// Counter to be used to figure out which sprite should be displayed
int charCounter = 7;
// Item to be used to check whether a string is being displayed
int ifStringItem = 123;
// First combo in the character list
int initCharCombo = 40000;
// Top left combo for the background
int initBGCombo = 39996;
int initBGCSet = 8;
// Position for the sprite
int spriteX = 40;
int spriteY = 120;
// Position for the background
int backX = 32;
int backY = 112;
while(true)
{
// If a String is being displayed
if(Link->Item[ifStringItem])
{
// Create the character FFC and display it
int character = Game->Counter[charCounter];
ffc char = Screen->LoadFFC(31);
char->Data = initCharCombo + character;
char->CSet = charCSet[character];
char->X = spriteX;
char->Y = spriteY;
// Create the char background FFC
ffc background = Screen->LoadFFC(32);
background->Data = initBGCombo;
background->CSet = initBGCSet;
background->TileHeight = 2;
background->TileWidth = 2;
background->X = backX;
background->Y = backY;
// Set the three FFCs to be above all
char->Flags[0] = true;
background->Flags[0] = true;
} // End of if(Link->Item[ifStringItem])
else
{
// Load the background and char FFCs
ffc char = Screen->LoadFFC(31);
ffc background = Screen->LoadFFC(32);
char->Data = 0;
background->Data = 0;
}
Waitframe();
} // End of while(true)
} // End of void run()
} // End of ffc script manageString
Example quest: http://www.uploading...essage.zip.html
Note that the combos for the character sprites start on combo 40,000. Also worthy of note is that you will have to edit some stuff, mostly the stuff right under void run() and before while(true). This is where the first character combo is, the location of the sprite on the screen, etc.
To give a very brief description of how it works once you have everything setup, you'll use string formatting to give Link the ifString item as well as set the character counter to the number of the character sprite you want displayed. This script will check if Link has that ifString item. If he does, then it'll find the correct sprite of that character and display it. Once you get done with the strings, you then remove the ifString item, removing the sprite and the background. Though, this can sometimes look weird if you don't put spaces after the actual text. Like I said, in the zip file theres a txt file that should help.
Edit: Nope! XD Here's the txt file. Sorry about that. http://www.uploading...essage.txt.html
Anyway, enjoy.

Edited by LinktheMaster, 07 July 2008 - 11:21 PM.