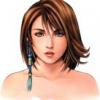
Harp of ages
#1
Posted 01 December 2008 - 08:29 PM
#2
Posted 01 December 2008 - 08:44 PM
Edited by lucas92, 01 December 2008 - 08:44 PM.
#3
Posted 01 December 2008 - 08:47 PM
Well im trying to script a harp of ages that requires to be a global script. Im thinking that it will require 3 flags: 1 for the portal , 1 to put on the entire floor when you obtain the tune of currents, and one to put of both the past and present when you have the tune of time. How do i set this up? Need ideas please.
A while back, I tried to make my own Harp of Ages. I really didn't get that far at all. The warping is INCREDIBLY hard when it comes to Tune of Currents/Ages, ESPECIALLY if your quest has a lot of events that force you onto a new map...
Here, let me give you some of my old code to start you off:
// Changable Variables:
// ID1- ID of the item you wish to be the Tune of Echoes, the first Harp tune. Use std.zh for the IDs.
// ID2- ID of the item you wish to be the Tune of Currents, the second Harp tune. See above.
// ID3- ID of the item you wish to be the Tune of Ages, the final Harp tune. Also see the first.
// DrmntTmeHle- Combo used by the Dormant/Sleeping Time Hole (Before using Tune of Echoes).
// OpnTmeHle- Combo used by the Open/Awakened Time Hole (After using Tune of Echoes).lp
import "std.zh"
item script HarpofAges
{
int CurMap = 0;
int CurDMap = 0;
int CurScrn = 0;
int LastMap = 0;
int LastDMap = 0;
int LastScrn = 0;
int WrpMap = 0;
int WrpDMap = 0;
int WrpScrn = 0;
int WrpMap2 = 0;
int WrpDMap2 = 0;
int WrpScrn2 = 0;
int ID1 = 32;
int ID2 = 55;
int ID3 = 0;
int DrmntTmeHle = 0;
int OpnTmeHle = 0;
int Time = 1;
int FFCLoad = 0;
int FFCLocation = 0;
void run()
{
CurMap = Game->GetCurMap();
CurDMap = Game->GetCurDMap();
CurScrn = Game->GetCurScreen();
WrpMap = CurMap + 1;
WrpDMap = CurDMap + 1;
WrpScrn = CurScrn;
WrpMap2 = CurMap - 1;
WrpDMap2 = CurDMap - 1;
WrpScrn2 = CurScrn;
if (Link->Item[ID3])
{
if (CurMap == 2 || CurMap == 4 || CurMap == 6 || CurMap == 8 || CurMap == 10)
{
Time = 0;
Link->PitWarp(WrpDMap2, WrpScrn2);
Quit();
}
else if (CurMap == 1 || CurMap == 3 || CurMap == 5 || CurMap == 7 || CurMap == 9)
{
Time = 1;
Link->PitWarp(WrpDMap, WrpScrn);
Quit();
}
}
else if (Link->Item[ID2])
{
if (CurMap == 2 || CurMap == 4 || CurMap == 6 || CurMap == 8 || CurMap == 10)
{
Time = 0;
Link->PitWarp(WrpDMap2, WrpScrn2);
Quit();
}
else if (CurMap == 1 || CurMap == 3 || CurMap == 5 || CurMap == 7 || CurMap == 9)
{
Time = 1;
}
}
else if (Link->Item[ID1])
{
ffc FFCLoad = Screen->LoadFFC(32);
FFCLocation = (FFCLoad->Y & 240)+(FFCLoad->X>>4);
if (Screen->ComboD [FFCLocation] == DrmntTmeHle)
{
Screen->ComboD [FFCLocation] = OpnTmeHle;
FFCLoad->Y = 0;
FFCLoad->X = 0;
}
}
}
}
Yeah, old stuff. However, you could make the code more efficient at checking for the right spot by using this snippet of code instead of the FFC checks:
{
if (Screen->ComboD[i] == InactiveTimeHole)
{
dostuff;
}
}
That would basically check every single combo on the screen for a certain combo, and when it finds the combo, it does whatever you tell it to.
This was one of my earliest scripts, so it may not look pretty. I never figured out why the script would never warp me when I had the Tune of Currents, so I gave up on it. You may continue off my code if you wish, or just use it as an example for making your own script. Either way, good luck! It won't be easy.
#4
Posted 01 December 2008 - 09:17 PM
#5
Posted 01 December 2008 - 09:20 PM
Anyway, just tried to help. I don't even know how that harp of ages work.

#6
Posted 01 December 2008 - 09:46 PM
#7
Posted 02 December 2008 - 03:11 AM
You do realise that'll mean you need 7 duplicate screens for every screen you make, right?
I thought about doing this for about a nano-second, then I realised that 3 duplicate screens for every screen way by far enough already.
#8
Posted 02 December 2008 - 04:15 AM

#9
Posted 02 December 2008 - 03:39 PM
Fair enough.
#10
Posted 02 December 2008 - 04:49 PM
#11
Posted 02 December 2008 - 04:59 PM
I just figured out i don't have patience writing in computer language. Now matter what i do to declare comboAt is undeclared. Also question. Is this valid?
Yes and no. You're wanting that to check if there's a combo at the location of the FFC "FFC", right? Well, this is where ComboAt comes in. It works as such:
ComboAt(location-x, location-y)
Simple. So, let's say we wanted to check for an FFC's location, and if a certain combo is there, then do stuff. You'd do this:
{
dostuff;
}
That's basically it. As for your warp... You're DEFINITELY using that wrong. Sadly, I forgot how to work those... I know it's simple, though. To set them up, at least.
#12
Posted 02 December 2008 - 05:03 PM
import "std.zh"
ComboAt is a function included in std.zh, so if you don't include that file, it won't work, unless you copy from std.zh that function.

#13
Posted 02 December 2008 - 09:47 PM
And if i check the activehole combo, and if true,can i make it an autowarp by using this code:
If(Screen->ComboD[ComboAt(this->X,this->Y)] ==CMB_ACTIVEHOLE){
this->Data = CMB_AUTOWARP;
Can I do that or do I need to check the combo type by using:
if(Screen->ComboT[combotypenumber] == CMB_ACTIVEHOLE);
this->Data = CMB_AUTOWARP;
Oh and the checking comes after the link animation is drawn and played.
Edited by drzchulo973, 03 December 2008 - 03:23 AM.
#14
Posted 03 December 2008 - 04:43 AM
You want the Harp of Ages and the Rod of Seasons in your quest?
You do realise that'll mean you need 7 duplicate screens for every screen you make, right?
I thought about doing this for about a nano-second, then I realised that 3 duplicate screens for every screen way by far enough already.
Why 7? What does the harp of ages do? ...I'm guessing it's something like time traveling.

...But wouldn't that still only require 3?
#15
Posted 03 December 2008 - 05:50 AM
Why 7? What does the harp of ages do? ...I'm guessing it's something like time traveling.

...But wouldn't that still only require 3?
Whoa that was tottally off topic from what I asked before you =p
But to answer your question, the harp of ages is used for time travel , as I am not adding the time traveling scene, because I'm not that too experienced to make something like that happen, even though I bet darkdragon would

And it doesn't require 3 duplicate maps and dmaps? Who said that? It only needs 2. That's why I'm using 2 flags.
0 user(s) are reading this topic
0 members, 0 guests, 0 anonymous users