CODE
ffc script lanterncombo
//Script for simulating a lanterns glow around link
//this script works! Note: It's possible to cheat by going to subscreen(it cancels the effect while bringing down the subscreen (if anyone can fix this, let me know))
//also, there maybe some flicker along the top tile, especially if link is moving fast, so best to limit motion to below those tiles.
//
//The way the script is setup, If you scroll onto the screen, theres a brief bit of darkness (60 frames).
//After that, if link has no item, the screen remains dark. If link has the "required item" he gets a small glow around him.
//Unless of course, he has the "Over rule item" in which case theres a small animation illuminating the entire screen.
//If you have one, and pick up the other while on screen, it will update your vision accordingly!
//Hence if you have the required, and pick up the over rule, the screen lights up!
//
//For some reason, it doesn't like text, or rather, text doesn't like it, because the text goes overwrites the script.
//Might have to do with what layer the darkness is on (currently layer 6)
//
//For this script, the TILES should be laid out like this:
//glow is currently a 3x3 square, but this can be altered to be bigger, but then you'll have to account for the Tdistance of the glow
//shadowtile= TL TC TR next animation frame...
// CL empty CR next animation frame...
// BL BC BR next animation frame...
//shadowtile2 = an 18x13 block of solid dark tiles, with an empty 3x3 spot missing from the bottom right corner WHICH MUST BE LEFT BLANK!
//lightup = a 1 x 4 row that is used to animate the light up gradient.
//It is important to note that these tiles must be placed before tile 32768, or, you need to edit the script to take that into account.
//Zquest will find the tile if its higher in value, but the inputs are signed floats which limits it to this value.
//
//Script by Xiion
{
void run(int shadowtile, int shadowtile2, int lightup, int item_req, int item_overrule, int cset, int torch_combo)
//shadowtile is the TILE number of the upper left tile of the 3x3 glow animation (see above)
//shadowtile2 is the TILE number of the upper left tile of the darkness block
//lightup is the left TILE of the light up animation
//item_req is the value of the item required to get a glow
//item_overrule is the value of the item required to null the darkness completely
//cset denotes which cset to use for the darkness
//torch_combo denotes the combo that represents a lit torch
{
int Tdistance = 0; //running value of the distance between link and the top of the screen
int initial_delay = 0;
if (initial_delay <= 60) //gives an inital darkness animation for the first 60 frames, while link scrolls across the screen
{
Tdistance = Ceiling(Div(Link->Y,16));//distance from link to subscreen in tiles
Screen->DrawTile(6, Link->X-1*16, Link->Y-1*16, shadowtile2, 3, 3, cset,1,0,0,0,0,1,128);
Screen->DrawTile(6, Link->X-16*16, Link->Y-Tdistance*16, shadowtile2+(11-Tdistance)*20, 18, Tdistance+3, cset,1,0,0,0,0,1,128); //Top left overlap
Screen->DrawTile(6, Link->X+2*16, Link->Y-Tdistance*16, shadowtile2+(11-Tdistance)*20, 15, Tdistance+3, cset,1,0,0,0,0,1,128); //Top right overlap
Screen->DrawTile(6, Link->X-16*16, Link->Y+2*16, shadowtile2, 18, 9, cset,1,0,0,0,0,1,128); //Bottom left overlap
Screen->DrawTile(6, Link->X+2*16, Link->Y+2*16, shadowtile2, 15, 9, cset,1,0,0,0,0,1,128); //Bottom right overlap
Screen->DrawTile(6, 0,0, shadowtile2, 16,1, cset,1,0,0,0,0,1,128); //top of screen overlap
initial_delay += 1;
Waitframe();
}
int max = lightup + 3; //gradient spans 4 tiles from lightup to lightup+3
int tile = 0;
int alreadylit = 0;
int x = -16;
int y = 0;
while(true)
{
//look for lit torches first
int torchnum =0;
int frames =0;
for (tile=0; tile<=175; tile++)
{
if (Screen->ComboD[tile] == torch_combo)
{
torchnum ++;
}
}
if (torchnum == 0)
{
alreadylit = 0;
}
if (torchnum >4)
{
torchnum=4;
}
if(Link->Item [item_overrule]) //if you have the overrule item, the screen lights up automatically
{
if (lightup <= max)
{
for(frames=0; frames<=4; frames++)
{
for (x=-16;x <= 256; x+=16)
{
for(y=0; y <= 176; y+=16)
{
Screen->DrawTile(6,x,y,lightup,1,1, cset,1,0,0,0,0,1,64);
}
}
Waitframe();
}
lightup++;
}
else
{
Waitframe();
}
}
else if(torchnum > 0 ) //if torches are lit, the screen will light up
{
for (x=-16;x <= 256; x+=16)
{
for(y=0; y <= 176; y+=16)
{
Screen->DrawTile(6,x,y,lightup+torchnum-1,1,1, cset,1,0,0,0,0,1,64);
}
}
Waitframe();
}
else if(Link->Item [item_req] && alreadylit==0) //if link only has the required item, and no torches are lit he gets a small animated glow around him
{
Tdistance = Ceiling(Div(Link->Y,16));//distance from link to subscreen in tiles
for(frames=0; frames<=4; frames++)
{
Screen->DrawTile(6, Link->X-1*16, Link->Y-1*16, shadowtile, 3, 3, cset,1,0,0,0,0,1,128);
Screen->DrawTile(6, Link->X-16*16, Link->Y-Tdistance*16, shadowtile2+(11-Tdistance)*20, 18, Tdistance+3, cset,1,0,0,0,0,1,128); //Top left overlap
Screen->DrawTile(6, Link->X+2*16, Link->Y-Tdistance*16, shadowtile2+(11-Tdistance)*20, 15, Tdistance+3, cset,1,0,0,0,0,1,128); //Top right overlap
Screen->DrawTile(6, Link->X-16*16, Link->Y+2*16, shadowtile2, 18, 9, cset,1,0,0,0,0,1,128); //Bottom left overlap
Screen->DrawTile(6, Link->X+2*16, Link->Y+2*16, shadowtile2, 15, 9, cset,1,0,0,0,0,1,128); //Bottom right overlap
Screen->DrawTile(6, 0,0, shadowtile2, 16,1, cset,1,0,0,0,0,1,128); //top of screen overlap
Waitframe();
}
for(frames=0; frames<=4; frames++)
{
Tdistance = Ceiling(Div(Link->Y,16));//distance from link to subscreen in tiles
Screen->DrawTile(6, Link->X-1*16, Link->Y-1*16, shadowtile+3, 3, 3, cset,1,0,0,0,0,1,128);
Screen->DrawTile(6, Link->X-16*16, Link->Y-Tdistance*16, shadowtile2+(11-Tdistance)*20, 18, Tdistance+3, cset,1,0,0,0,0,1,128); //Top left overlap
Screen->DrawTile(6, Link->X+2*16, Link->Y-Tdistance*16, shadowtile2+(11-Tdistance)*20, 15, Tdistance+3, cset,1,0,0,0,0,1,128); //Top right overlap
Screen->DrawTile(6, Link->X-16*16, Link->Y+2*16, shadowtile2, 18, 9, cset,1,0,0,0,0,1,128); //Bottom left overlap
Screen->DrawTile(6, Link->X+2*16, Link->Y+2*16, shadowtile2, 15, 9, cset,1,0,0,0,0,1,128); //Bottom right overlap
Screen->DrawTile(6, 0,0, shadowtile2, 16,1, cset,1,0,0,0,0,1,128); //top of screen overlap
Waitframe();
}
for(frames=0; frames<=4; frames++)
{
Tdistance = Ceiling(Div(Link->Y,16));//distance from link to subscreen in tiles
Screen->DrawTile(6, Link->X-1*16, Link->Y-1*16, shadowtile+6, 3, 3, cset,1,0,0,0,0,1,128);
Screen->DrawTile(6, Link->X-16*16, Link->Y-Tdistance*16, shadowtile2+(11-Tdistance)*20, 18, Tdistance+3, cset,1,0,0,0,0,1,128); //Top left overlap
Screen->DrawTile(6, Link->X+2*16, Link->Y-Tdistance*16, shadowtile2+(11-Tdistance)*20, 15, Tdistance+3, cset,1,0,0,0,0,1,128); //Top right overlap
Screen->DrawTile(6, Link->X-16*16, Link->Y+2*16, shadowtile2, 18, 9, cset,1,0,0,0,0,1,128); //Bottom left overlap
Screen->DrawTile(6, Link->X+2*16, Link->Y+2*16, shadowtile2, 15, 9, cset,1,0,0,0,0,1,128); //Bottom right overlap
Screen->DrawTile(6, 0,0, shadowtile2, 16,1, cset,1,0,0,0,0,1,128); //top of screen overlap
Waitframe();
}
for(frames=0; frames<=4; frames++)
{
Tdistance = Ceiling(Div(Link->Y,16));//distance from link to subscreen in tiles
Screen->DrawTile(6, Link->X-1*16, Link->Y-1*16, shadowtile+3, 3, 3, cset,1,0,0,0,0,1,128);
Screen->DrawTile(6, Link->X-16*16, Link->Y-Tdistance*16, shadowtile2+(11-Tdistance)*20, 18, Tdistance+3, cset,1,0,0,0,0,1,128); //Top left overlap
Screen->DrawTile(6, Link->X+2*16, Link->Y-Tdistance*16, shadowtile2+(11-Tdistance)*20, 15, Tdistance+3, cset,1,0,0,0,0,1,128); //Top right overlap
Screen->DrawTile(6, Link->X-16*16, Link->Y+2*16, shadowtile2, 18, 9, cset,1,0,0,0,0,1,128); //Bottom left overlap
Screen->DrawTile(6, Link->X+2*16, Link->Y+2*16, shadowtile2, 15, 9, cset,1,0,0,0,0,1,128); //Bottom right overlap
Screen->DrawTile(6, 0,0, shadowtile2, 16,1, cset,1,0,0,0,0,1,128); //top of screen overlap
Waitframe();
}
}
else //if link has neither item and torches aren't lit, the screen remains blank
{
Tdistance = Ceiling(Div(Link->Y,16));//distance from link to subscreen in tiles
Screen->DrawTile(6, Link->X-1*16, Link->Y-1*16, shadowtile2, 3, 3, cset,1,0,0,0,0,1,128);
Screen->DrawTile(6, Link->X-16*16, Link->Y-Tdistance*16, shadowtile2+(11-Tdistance)*20, 18, Tdistance+3, cset,1,0,0,0,0,1,128); //Top left overlap
Screen->DrawTile(6, Link->X+2*16, Link->Y-Tdistance*16, shadowtile2+(11-Tdistance)*20, 15, Tdistance+3, cset,1,0,0,0,0,1,128); //Top right overlap
Screen->DrawTile(6, Link->X-16*16, Link->Y+2*16, shadowtile2, 18, 9, cset,1,0,0,0,0,1,128); //Bottom left overlap
Screen->DrawTile(6, Link->X+2*16, Link->Y+2*16, shadowtile2, 15, 9, cset,1,0,0,0,0,1,128); //Bottom right overlap
Screen->DrawTile(6, 0,0, shadowtile2, 16,1, cset,1,0,0,0,0,1,128); //top of screen overlap
Waitframe();
}
}
}
}
//Script for simulating a lanterns glow around link
//this script works! Note: It's possible to cheat by going to subscreen(it cancels the effect while bringing down the subscreen (if anyone can fix this, let me know))
//also, there maybe some flicker along the top tile, especially if link is moving fast, so best to limit motion to below those tiles.
//
//The way the script is setup, If you scroll onto the screen, theres a brief bit of darkness (60 frames).
//After that, if link has no item, the screen remains dark. If link has the "required item" he gets a small glow around him.
//Unless of course, he has the "Over rule item" in which case theres a small animation illuminating the entire screen.
//If you have one, and pick up the other while on screen, it will update your vision accordingly!
//Hence if you have the required, and pick up the over rule, the screen lights up!
//
//For some reason, it doesn't like text, or rather, text doesn't like it, because the text goes overwrites the script.
//Might have to do with what layer the darkness is on (currently layer 6)
//
//For this script, the TILES should be laid out like this:
//glow is currently a 3x3 square, but this can be altered to be bigger, but then you'll have to account for the Tdistance of the glow
//shadowtile= TL TC TR next animation frame...
// CL empty CR next animation frame...
// BL BC BR next animation frame...
//shadowtile2 = an 18x13 block of solid dark tiles, with an empty 3x3 spot missing from the bottom right corner WHICH MUST BE LEFT BLANK!
//lightup = a 1 x 4 row that is used to animate the light up gradient.
//It is important to note that these tiles must be placed before tile 32768, or, you need to edit the script to take that into account.
//Zquest will find the tile if its higher in value, but the inputs are signed floats which limits it to this value.
//
//Script by Xiion
{
void run(int shadowtile, int shadowtile2, int lightup, int item_req, int item_overrule, int cset, int torch_combo)
//shadowtile is the TILE number of the upper left tile of the 3x3 glow animation (see above)
//shadowtile2 is the TILE number of the upper left tile of the darkness block
//lightup is the left TILE of the light up animation
//item_req is the value of the item required to get a glow
//item_overrule is the value of the item required to null the darkness completely
//cset denotes which cset to use for the darkness
//torch_combo denotes the combo that represents a lit torch
{
int Tdistance = 0; //running value of the distance between link and the top of the screen
int initial_delay = 0;
if (initial_delay <= 60) //gives an inital darkness animation for the first 60 frames, while link scrolls across the screen
{
Tdistance = Ceiling(Div(Link->Y,16));//distance from link to subscreen in tiles
Screen->DrawTile(6, Link->X-1*16, Link->Y-1*16, shadowtile2, 3, 3, cset,1,0,0,0,0,1,128);
Screen->DrawTile(6, Link->X-16*16, Link->Y-Tdistance*16, shadowtile2+(11-Tdistance)*20, 18, Tdistance+3, cset,1,0,0,0,0,1,128); //Top left overlap
Screen->DrawTile(6, Link->X+2*16, Link->Y-Tdistance*16, shadowtile2+(11-Tdistance)*20, 15, Tdistance+3, cset,1,0,0,0,0,1,128); //Top right overlap
Screen->DrawTile(6, Link->X-16*16, Link->Y+2*16, shadowtile2, 18, 9, cset,1,0,0,0,0,1,128); //Bottom left overlap
Screen->DrawTile(6, Link->X+2*16, Link->Y+2*16, shadowtile2, 15, 9, cset,1,0,0,0,0,1,128); //Bottom right overlap
Screen->DrawTile(6, 0,0, shadowtile2, 16,1, cset,1,0,0,0,0,1,128); //top of screen overlap
initial_delay += 1;
Waitframe();
}
int max = lightup + 3; //gradient spans 4 tiles from lightup to lightup+3
int tile = 0;
int alreadylit = 0;
int x = -16;
int y = 0;
while(true)
{
//look for lit torches first
int torchnum =0;
int frames =0;
for (tile=0; tile<=175; tile++)
{
if (Screen->ComboD[tile] == torch_combo)
{
torchnum ++;
}
}
if (torchnum == 0)
{
alreadylit = 0;
}
if (torchnum >4)
{
torchnum=4;
}
if(Link->Item [item_overrule]) //if you have the overrule item, the screen lights up automatically
{
if (lightup <= max)
{
for(frames=0; frames<=4; frames++)
{
for (x=-16;x <= 256; x+=16)
{
for(y=0; y <= 176; y+=16)
{
Screen->DrawTile(6,x,y,lightup,1,1, cset,1,0,0,0,0,1,64);
}
}
Waitframe();
}
lightup++;
}
else
{
Waitframe();
}
}
else if(torchnum > 0 ) //if torches are lit, the screen will light up
{
for (x=-16;x <= 256; x+=16)
{
for(y=0; y <= 176; y+=16)
{
Screen->DrawTile(6,x,y,lightup+torchnum-1,1,1, cset,1,0,0,0,0,1,64);
}
}
Waitframe();
}
else if(Link->Item [item_req] && alreadylit==0) //if link only has the required item, and no torches are lit he gets a small animated glow around him
{
Tdistance = Ceiling(Div(Link->Y,16));//distance from link to subscreen in tiles
for(frames=0; frames<=4; frames++)
{
Screen->DrawTile(6, Link->X-1*16, Link->Y-1*16, shadowtile, 3, 3, cset,1,0,0,0,0,1,128);
Screen->DrawTile(6, Link->X-16*16, Link->Y-Tdistance*16, shadowtile2+(11-Tdistance)*20, 18, Tdistance+3, cset,1,0,0,0,0,1,128); //Top left overlap
Screen->DrawTile(6, Link->X+2*16, Link->Y-Tdistance*16, shadowtile2+(11-Tdistance)*20, 15, Tdistance+3, cset,1,0,0,0,0,1,128); //Top right overlap
Screen->DrawTile(6, Link->X-16*16, Link->Y+2*16, shadowtile2, 18, 9, cset,1,0,0,0,0,1,128); //Bottom left overlap
Screen->DrawTile(6, Link->X+2*16, Link->Y+2*16, shadowtile2, 15, 9, cset,1,0,0,0,0,1,128); //Bottom right overlap
Screen->DrawTile(6, 0,0, shadowtile2, 16,1, cset,1,0,0,0,0,1,128); //top of screen overlap
Waitframe();
}
for(frames=0; frames<=4; frames++)
{
Tdistance = Ceiling(Div(Link->Y,16));//distance from link to subscreen in tiles
Screen->DrawTile(6, Link->X-1*16, Link->Y-1*16, shadowtile+3, 3, 3, cset,1,0,0,0,0,1,128);
Screen->DrawTile(6, Link->X-16*16, Link->Y-Tdistance*16, shadowtile2+(11-Tdistance)*20, 18, Tdistance+3, cset,1,0,0,0,0,1,128); //Top left overlap
Screen->DrawTile(6, Link->X+2*16, Link->Y-Tdistance*16, shadowtile2+(11-Tdistance)*20, 15, Tdistance+3, cset,1,0,0,0,0,1,128); //Top right overlap
Screen->DrawTile(6, Link->X-16*16, Link->Y+2*16, shadowtile2, 18, 9, cset,1,0,0,0,0,1,128); //Bottom left overlap
Screen->DrawTile(6, Link->X+2*16, Link->Y+2*16, shadowtile2, 15, 9, cset,1,0,0,0,0,1,128); //Bottom right overlap
Screen->DrawTile(6, 0,0, shadowtile2, 16,1, cset,1,0,0,0,0,1,128); //top of screen overlap
Waitframe();
}
for(frames=0; frames<=4; frames++)
{
Tdistance = Ceiling(Div(Link->Y,16));//distance from link to subscreen in tiles
Screen->DrawTile(6, Link->X-1*16, Link->Y-1*16, shadowtile+6, 3, 3, cset,1,0,0,0,0,1,128);
Screen->DrawTile(6, Link->X-16*16, Link->Y-Tdistance*16, shadowtile2+(11-Tdistance)*20, 18, Tdistance+3, cset,1,0,0,0,0,1,128); //Top left overlap
Screen->DrawTile(6, Link->X+2*16, Link->Y-Tdistance*16, shadowtile2+(11-Tdistance)*20, 15, Tdistance+3, cset,1,0,0,0,0,1,128); //Top right overlap
Screen->DrawTile(6, Link->X-16*16, Link->Y+2*16, shadowtile2, 18, 9, cset,1,0,0,0,0,1,128); //Bottom left overlap
Screen->DrawTile(6, Link->X+2*16, Link->Y+2*16, shadowtile2, 15, 9, cset,1,0,0,0,0,1,128); //Bottom right overlap
Screen->DrawTile(6, 0,0, shadowtile2, 16,1, cset,1,0,0,0,0,1,128); //top of screen overlap
Waitframe();
}
for(frames=0; frames<=4; frames++)
{
Tdistance = Ceiling(Div(Link->Y,16));//distance from link to subscreen in tiles
Screen->DrawTile(6, Link->X-1*16, Link->Y-1*16, shadowtile+3, 3, 3, cset,1,0,0,0,0,1,128);
Screen->DrawTile(6, Link->X-16*16, Link->Y-Tdistance*16, shadowtile2+(11-Tdistance)*20, 18, Tdistance+3, cset,1,0,0,0,0,1,128); //Top left overlap
Screen->DrawTile(6, Link->X+2*16, Link->Y-Tdistance*16, shadowtile2+(11-Tdistance)*20, 15, Tdistance+3, cset,1,0,0,0,0,1,128); //Top right overlap
Screen->DrawTile(6, Link->X-16*16, Link->Y+2*16, shadowtile2, 18, 9, cset,1,0,0,0,0,1,128); //Bottom left overlap
Screen->DrawTile(6, Link->X+2*16, Link->Y+2*16, shadowtile2, 15, 9, cset,1,0,0,0,0,1,128); //Bottom right overlap
Screen->DrawTile(6, 0,0, shadowtile2, 16,1, cset,1,0,0,0,0,1,128); //top of screen overlap
Waitframe();
}
}
else //if link has neither item and torches aren't lit, the screen remains blank
{
Tdistance = Ceiling(Div(Link->Y,16));//distance from link to subscreen in tiles
Screen->DrawTile(6, Link->X-1*16, Link->Y-1*16, shadowtile2, 3, 3, cset,1,0,0,0,0,1,128);
Screen->DrawTile(6, Link->X-16*16, Link->Y-Tdistance*16, shadowtile2+(11-Tdistance)*20, 18, Tdistance+3, cset,1,0,0,0,0,1,128); //Top left overlap
Screen->DrawTile(6, Link->X+2*16, Link->Y-Tdistance*16, shadowtile2+(11-Tdistance)*20, 15, Tdistance+3, cset,1,0,0,0,0,1,128); //Top right overlap
Screen->DrawTile(6, Link->X-16*16, Link->Y+2*16, shadowtile2, 18, 9, cset,1,0,0,0,0,1,128); //Bottom left overlap
Screen->DrawTile(6, Link->X+2*16, Link->Y+2*16, shadowtile2, 15, 9, cset,1,0,0,0,0,1,128); //Bottom right overlap
Screen->DrawTile(6, 0,0, shadowtile2, 16,1, cset,1,0,0,0,0,1,128); //top of screen overlap
Waitframe();
}
}
}
}
If you find a bug, let me know I'll see what I can do to fix it.
Yeah... its a bit lengthy (not as much now!), but it works. But if you can simplify it... by all means, please let me know....
Edit: Now with Torch compatibility

Unfortunately... when the torches are lit, it does slow down zquest noticeably. My best guess as to why this is, it's because of the drawtiles translucency. I don't see anything else that could be doing it. Maybe someone smarter than me can see some other problem.
To setup the tiles for this combo:
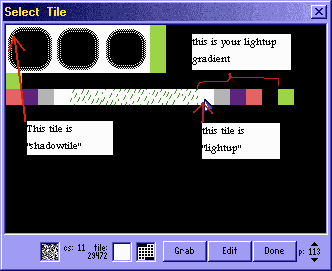
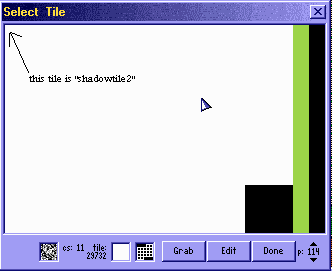
*Note: For convenience, I used a full color scheme to show all the tiles. In the images, Black is the transparent color.
On the images, I've pointed out the tiles that are referenced by the ffc arguments. Order and placement of the groups of tiles aren't important, but it is important that they stay as a group. (You can ignore the "rain" tiles to the left of the "lightup" tile, they were/are for something else, i just wanted to use the gradient there)
Shadowtile is the top left of the 1st of a 4 frame animation (1-2-3-2) with the sequential frames adjacent to the right.
Lightup is the darkest portion of 4* color gradient. *The code says its 4, my math says its 4, but for some reason, it likes 5 there. Yeah, I don't get it either

Shadowtile 2 is the top left tile of the big patch of darkness. This is an 18x13 block of tiles. It is very important that the bottom right block of 3x3 remains transparent! That block MUST REMAIN EMPTY! Otherwise you'll cover up your animation! I'm serious! Only cause I've made that mistake a dozen times, and its annoyed me everytime

Edited by Xiion, 06 July 2008 - 09:38 PM.