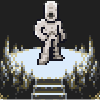
Ice combos.
#1
Posted 01 September 2011 - 02:26 PM
To be honest, I really don't care how its done, or how messy the code is (Since you would have to edit Link's X and Y because there is no Link->Vx, etc.) as long as its condensed into a function that can be placed in the while() loop of my global script.
Can someone do this for me? I have no idea how I'd go about doing this.
#2
Posted 18 September 2011 - 10:38 AM
import "ffcscript.zh"
const int CT_ICE2 = 142;
const int SCRIPT_ICECOMBO = 1;
bool OnIce(){
int combo = ComboAt(CenterLinkX(), CenterLinkY());
return(Screen->ComboT[combo] == CT_ICE2 && Link->Z == 0);
}
global script Slot2{
void run(){
while(true){
if(OnIce() && CountFFCsRunning(SCRIPT_ICECOMBO) == 0 && Link->Action == LA_WALKING){
int args[8];
RunFFCScript(SCRIPT_ICECOMBO, args);
}
Waitframe();
}
}
}
ffc script IceCombo{
void run(){
this->X = Link->X;
this->Y = Link->Y;
int PreviousInput = DirInput();
int PreviousDir = Link->Dir;
Waitdraw();
while(PreviousInput == DirInput() && PreviousDir == Link->Dir){
if(!OnIce()) Quit();
PreviousInput = DirInput();
PreviousDir = Link->Dir;
Waitframe();
}
int dir = AngleDir8(Angle(this->X, this->Y, Link->X, Link->Y));
float xstep;
float ystep;
for(int i = 100; i > 0; i--){
if(!OnIce()) break;
if(dir == 0 || dir == 4 || dir == 5) ystep -= i/100;
else if(dir == 1 || dir == 6 || dir == 7) ystep += i/100;
if(dir == 2 || dir == 4 || dir == 6) xstep -= i/100;
else if(dir == 3 || dir == 5 || dir == 7) xstep += i/100;
if(xstep <= -1 || xstep >= 1){
if(xstep < 0 && CanWalk(Link->X, Link->Y, DIR_LEFT, Abs(xstep), false)) Link->X += xstep;
else if(xstep > 0 && CanWalk(Link->X, Link->Y, DIR_RIGHT, xstep, false)) Link->X += xstep;
xstep = 0;
}
if(ystep <= -1 || ystep >= 1){
if(ystep < 0 && CanWalk(Link->X, Link->Y, DIR_UP, Abs(ystep), false)) Link->Y += xstep;
else if(ystep > 0 && CanWalk(Link->X, Link->Y, DIR_DOWN, ystep, false)) Link->Y += xstep;
ystep = 0;
}
Waitframe();
}
}
int DirInput(){
int bit;
if(Link->InputUp) bit |= 1b;
if(Link->InputDown) bit |= 10b;
if(Link->InputLeft) bit |= 100b;
if(Link->InputRight) bit |= 1000b;
return bit;
}
}
Let me know if this works for you or if you need help setting it up.
#3
Posted 26 September 2011 - 11:12 PM
#4
Posted 28 September 2011 - 10:07 AM
#5
Posted 28 September 2011 - 12:07 PM
Erm... Alright, I see its in 2 pieces. Do I need to place the FFC script on every screen that has "Ice combos"? Or does the script handle that?
No, it only work in RC2 I forgot to mention that, and you'll need saffiths ffcscript.zh. The globalscript will launch the ice combo script as needed. Just be sure the ffc script num of the ice combo is the same as the script and CT_ICE2 = the value of CT_SCRPT# "whatever scripted combo you want to use for ice that is" So no, you don't need to put the ice combo on every screen that has ice combos. The global script does that for you and will launch a script when you're waling on Ice. Make sense?
#6
Posted 28 September 2011 - 03:17 PM

Edit: Any way to merge it with your Ice Arrow script? because (global) slot2 is used in both. I know there was a tutorial about it but i don't get how it works.
Edited by Gonken, 28 September 2011 - 03:23 PM.
#7
Posted 28 September 2011 - 03:25 PM
int args[8];
RunFFCScript(SCRIPT_ICECOMBO, args);
}
Waitframe();
Once the global section is done, paste the part of the code starting with "ffc script ice combo" into the bottom of your script file.
Edited by MoscowModder, 28 September 2011 - 03:27 PM.
#8
Posted 28 September 2011 - 03:32 PM

Should i keep the waitframe thing and should i remove the bool OnIce() from the ice combo script?
#9
Posted 28 September 2011 - 03:52 PM
#10
Posted 28 September 2011 - 03:54 PM

Edit: It executed succesfully without removing waitframe, the Slot2 looks like this: (and there is a waitframe in the beginning and at the end)
void run(){
while(true){
if(OnIce() && CountFFCsRunning(SCRIPT_ICECOMBO) == 0 && Link->Action == LA_WALKING){
int args[8];
RunFFCScript(SCRIPT_ICECOMBO, args);
}
Waitframe();
if(icelevel != 0){
for(int w = Screen->NumLWeapons(); w > 0; w--){
lweapon wpn = Screen->LoadLWeapon(w);
if(wpn->isValid()){
if(wpn->ID == LW_SPECIALARROW && wpn->Misc[3] == 2){
npc enemy;
if(HitEdge(wpn)) wpn->Misc[1] = 2;
for(int e = Screen->NumNPCs(); e > 0; e--){
enemy = Screen->LoadNPC(e);
if(Collision(wpn, enemy)){
wpn->Misc[1] = 1;
break;
}
}
if(wpn->Misc[1] != 0){
if(icelevel == 1){
if(wpn->Misc[1] == 1) Freeze(enemy);
}
else if(icelevel == 2){
if(wpn->Misc[1] == 1) Freeze(enemy);
for(int i = 4; i < 8; i += 1){
lweapon shard = CreateLWeaponAt(LW_SHARD,wpn->X,wpn->Y);
shard->UseSprite(WPS_ICESHARD);
shard->Dir = i;
shard->Step = 300;
if(shard->Dir == 4) shard->Flip = 0;
else if(shard->Dir == 5) shard->Flip = 1;
else if(shard->Dir == 6) shard->Flip = 2;
else if(shard->Dir == 7) shard->Flip = 3;
shard->Damage = wpn->Misc[0];
}
}
wpn->DeadState = WDS_DEAD;
}
else if(wpn->Misc[2] % 4 == 0){
lweapon sparkle = CreateLWeaponAt(LW_SPARKLE,wpn->X,wpn->Y);
sparkle->UseSprite(WPS_ICESPARKLE);
}
wpn->Misc[2]++;
}
}
}
}
Waitframe();
}
}
void Freeze(npc enemy){
if(NumNPCsOf(NPC_FREEZER) == 0) CreateNPCAt(NPC_FREEZER,0,0);
if(enemy->Attributes[11] != 1){
enemy->Stun = -1;
enemy->CSet = 14;
}
}
}
Edited by Gonken, 28 September 2011 - 04:01 PM.
#11
Posted 28 September 2011 - 04:08 PM
#13
Posted 28 September 2011 - 07:07 PM
EDIT: It works. Sort of.
Movement is sort of wierd though. Sometimes Link simply doesn't slide. Sometimes he slides wayyyy too fast after changing directions. But it seems sort of random...
Might be a bug with non-diagonal movement though
EDIT: Not a bug with diagonal movement. Seems that Link cannot slide in more than one direction. If he's sliding when I change direction, he will continue sliding in the first direction and will not slide in any other direction.
Edited by Master Maniac, 28 September 2011 - 07:28 PM.
#14
Posted 29 September 2011 - 09:44 PM
if(ystep <= -1 || ystep >= 1){
if(ystep < 0 && CanWalk(Link->X, Link->Y, DIR_UP, Abs(ystep), false)) Link->Y += xstep;
else if(ystep > 0 && CanWalk(Link->X, Link->Y, DIR_DOWN, ystep, false)) Link->Y += xstep;
ystep = 0;
I think BB meant for the 2 instances of xstep in this segment to be ystep. Try that and see if it helps.
#15
Posted 16 October 2011 - 12:31 PM
0 user(s) are reading this topic
0 members, 0 guests, 0 anonymous users