So, basically, I'm looking for a way to reduce the number on one of the counters (I'll be using CR_SCRIPT1 for this counter, if it matters) by 1, every minute or so (360 frames, if I'm not mistaken), but I'm not finding what I'm looking for in any of the documentation or forums, perhaps because I am not looking correctly. Can anyone point me to what I'm looking for?
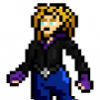
Periodic reduction to a counter
#1
Posted 15 December 2014 - 12:59 PM
#2
Posted 15 December 2014 - 01:14 PM
#3
Posted 15 December 2014 - 01:17 PM
Yeah, I get that part, what I'm not understanding is how to A. Reference the number of items represented by the counter (for example, if I have 10 arrows and I want something to happen specifically when there are 10 arrows), and B. reduce that number by 1 every 360 frames
#4
Posted 15 December 2014 - 01:22 PM
for (int i = 0; true; i = (i + 1) % 360) { if (i == 0 && Game->Counter[CR_ARROWS] >= 10) { Game->Counter[CR_SCRIPT1]--; } Waitframe(); }
A for loop like this is functionally equivalent to a while loop, but with a counter you can reference for stuff like this. You can also declare another variable and use it in a while loop. Lots of ways to accomplish the same thing.
#5
Posted 15 December 2014 - 01:37 PM
So.... To accomplish what I'm going for (the ability for Link to get progressively drunker,depending on how many beers he's had), it should look something like this?
void run() { for (int i = 0; true; i = (i+1) % 360) if(i == 00 && Game->Counter[CR_SCRIPT1] >= 1 ){ Link->Drunk = i*360; } Waitframe(); } } }
Or did I screw something up? I'm still not quite understanding what variable I'm using to reference the number of items in the scripted counter.
#6
Posted 15 December 2014 - 02:53 PM
I'm still not quite understanding what variable I'm using to reference the number of items in the scripted counter.
the counter is just keeping track of how many of that item you have - if arrows its how many arrows. not how many items use that counter, for instance if you had two different bows using that counter.
based on your code, you are using the counter for number of beers consumed? and that makes Link drunker?
in Lejes example code, every 360 frames the Script1 counter is being reduced by 1 if Link has over 10 arrows (CR_ARROW counter).
in your code every 360 frames in Script1 is more than 1, Link is drunker. But not really because for the if statement to be true i=0, and then you are multiplying i ( or zero) by 360 and assigning it to Link->Drunk, so Link is never drunk.
you probably want your drunk code to be
Link->Drunk = Game->Counter[CR_SCRIPT1] *360;
that will make the Drunk effect last for 360 frames times how many of the counter (beers) he's had...
#7
Posted 15 December 2014 - 03:16 PM
You know what? I think this just got too confusing for me. Is there a way to just reset the "beer" count to 0 when the drunk effect finishes? since the built-in effect reduces in severity as it goes down, it would probably be cleaner to just let it run down the timer, something like this, maybe? (Yeah, I have no idea what I'm doing.)
void run() { //Drunk script while (true) if (Game->Counter[CR_SCRIPT1] >= 1 ){ Link->Drunk = Game->Counter[CR_SCRIPT1] * 360; { if (Link->Drunk == 0) { Game->Counter[CR_SCRIPT1] = 0; } } } Waitframe(); } } }
#8
Posted 15 December 2014 - 05:26 PM
that looks good, except that your first if statement is going to keep making Link drunk, so drunk will never become 0 to make the next if statement trigger, and reset the Script counter.
something like this. it would be a global script. just put DrunkScript(); somewhere before the Waitframe(); in your global loop.
void DrunkScript(){ if (Game->Counter[CR_SCRIPT1] > 0 ){ Link->Drunk = Game->Counter[CR_SCRIPT1] * 360; CR_SCRIPT1 = 0; } }
#9
Posted 15 December 2014 - 05:41 PM
Well, that looks nice. Now I just have to figure out how to permanently reassign InputL and InputR to either InputLeft and InputRight or to disable them completely
#10
Posted 15 December 2014 - 05:56 PM
this might work, not sure if the Drunk effect is actually triggering InputL/R so it might not work. your better solution would be to use the confusion script that Lejes (?) linked in the other thread.
void DrunkScript(){ if (Game->Counter[CR_SCRIPT1] > 0 ){ Link->Drunk = Game->Counter[CR_SCRIPT1] * 360; CR_SCRIPT1 = 0; } if(Link->Drunk>0){ Link->InputL = false; Link->InputR = false; } }
#11
Posted 15 December 2014 - 06:00 PM
Well, it's compiling, all right, but it doesn't seem to do anything. The counter increases, but Link just isn't getting drunk.
#12
Posted 15 December 2014 - 06:51 PM
void DrunkScript(){ if (Game->Counter[CR_SCRIPT1] > 0 ){ Link->Drunk = Game->Counter[CR_SCRIPT1] * 360; //CR_SCRIPT1 = 0; //This is the problem Game->Counter[CR_SCRIPT1]=0; } if(Link->Drunk>0){ Link->InputL = false; Link->InputR = false; } }
a minute is 60*60 frames that's 3600 btw.
Also Link Drunk is reduced by 1 every frame.
Edited by Freya, 15 December 2014 - 06:54 PM.
#13
Posted 15 December 2014 - 06:59 PM
//CR_SCRIPT1 = 0; //This is the problem
whoops! yup, that would be the problem. interesting how that wouldn't create a compile error.
#14
Posted 15 December 2014 - 08:19 PM
It did, I changed it to
Game->Counter[CR_SCRIPT1] = 0
to get it to compile. But... it still doesn't do anything. Even got 10 beers, all set to increase the Script 1 counter by 1, and no drunk effect, whatsoever. Also, I changed the 360 to 3600, since, yeah, I totally screwed my math on that one.
global script Slot1 { //Drunk script void run(){ if (Game->Counter[CR_SCRIPT1] > 0 ){ Link->Drunk = Game->Counter[CR_SCRIPT1] * 3600; Game->Counter[CR_SCRIPT1] = 0; } if(Link->Drunk>0){ Link->InputL = false; Link->InputR = false; } Waitframe(); } }
Also, it wouldn't compile until I changed the "void DrunkScript()" to "void run()"
Edited by Lineas, 15 December 2014 - 08:21 PM.
#15
Posted 15 December 2014 - 08:24 PM
Wait... why is this called slot1?
If you want it to happen continuously you need to use slot2 and put it in a while() loop
Edited by Freya, 15 December 2014 - 08:25 PM.
0 user(s) are reading this topic
0 members, 0 guests, 0 anonymous users